Table Of Content
Three of the header files (complex.h, stdatomic.h, and threads.h) are conditional features that implementations are not required to support. This concludes our first part, and we merely scratched the surface of possibilities we have with pointers, which just shows how complex the seemingly simple concept of “it’s just a memory address” can really be. Next time, we continue with pointer arithmetic and some more complex pointer arrangements. This is a perfectly valid cast, and as long as accessing members of bar won’t go beyond the size of foo, we will be on the safe side. Whether such a type cast makes sense or not is of course a different story, and depends on the context, but pointers give us the freedom to cast as we please.
Bitwise OR
In 1982, Stroustrup started to develop a successor to C with Classes, which he named "C++" (++ being the increment operator in C) after going through several other names. New features were added, including virtual functions, function name and operator overloading, references, constants, type-safe free-store memory allocation (new/delete), improved type checking, and BCPL-style single-line comments with two forward slashes (//). Furthermore, Stroustrup developed a new, standalone compiler for C++, Cfront.
C Sharp (programming language)
Objects declared outside of all blocks and those explicitly declared with the static storage class specifier have static storage duration. If signed or unsigned is not specified explicitly, in most circumstances, signed is assumed. However, for historic reasons, plain char is a type distinct from both signed char and unsigned char. It may be a signed type or an unsigned type, depending on the compiler and the character set (C guarantees that members of the C basic character set have positive values).
Other languages
This work culminated in the creation of the so-called C89 standard in 1989. Part of the resulting standard was a set of software libraries called the ANSI C standard library. The original C language provided no built-in functions such as I/O operations, unlike traditional languages such as COBOL and Fortran.[citation needed] Over time, user communities of C shared ideas and implementations of what is now called C standard libraries. Many of these ideas were incorporated eventually into the definition of the standardized C language. The char type is distinct from both signed char and unsigned char, but is guaranteed to have the same representation as one of them.
A number of other groups are using other nonstandard headers – the GNU C Library has alloca.h, and OpenVMS has the va_count() function. The most important requirement to succeed with pointers and avoid segmentation faults is that they always have a large enough place inside memory that they can actually access. Again, on a system without MMU, any location in the memory is technically such a place, but it’s a lot stricter with memory protection, and we also need to avoid pointers that don’t point to anywhere specific. So if you’ve always been a little fuzzy on pointers, read on for some real-world scenarios of where and how pointers are used.
Operator precedence
Code that is not marked as unsafe can still store and manipulate pointers through the System.IntPtr type, but it cannot dereference them. Ordinarily, when a function in a derived class overrides a function in a base class, the function to call is determined by the type of the object. A given function is overridden when there exists no difference in the number or type of parameters between two or more definitions of that function. Hence, at compile time, it may not be possible to determine the type of the object and therefore the correct function to call, given only a base class pointer; the decision is therefore put off until runtime.

Local use-after-free bugs are usually easy for static analyzers to recognize. Therefore, this approach is less useful for local pointers and it is more often used with pointers stored in long-living structs. In general though, setting pointers to NULL is good practice [according to whom? ] as it allows a programmer to NULL-check pointers prior to dereferencing, thus helping prevent crashes. An incomplete type is a structure or union type whose members have not yet been specified, an array type whose dimension has not yet been specified, or the void type (the void type cannot be completed). Such a type may not be instantiated (its size is not known), nor may its members be accessed (they, too, are unknown); however, the derived pointer type may be used (but not dereferenced).
Data types
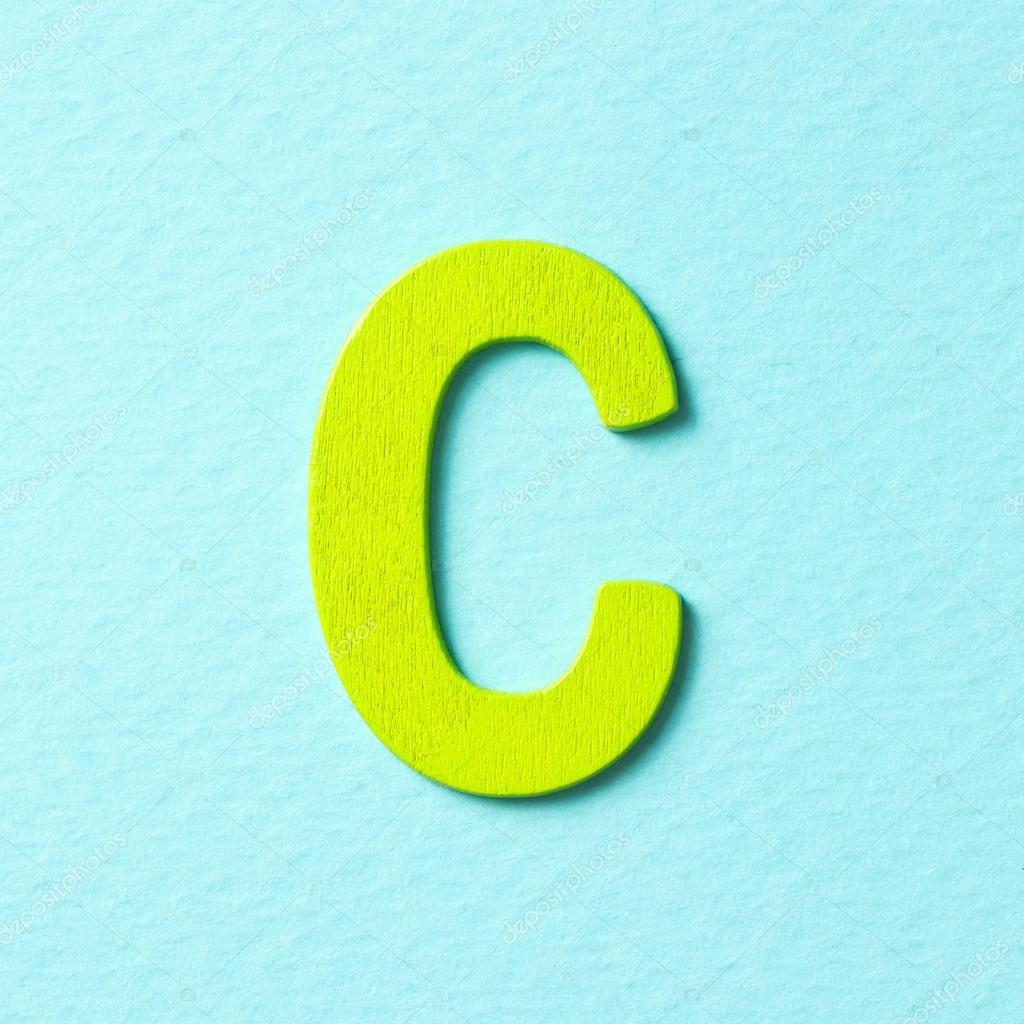
For example, the Console class used later in the source code is defined in the System namespace, meaning it can be used without supplying the full name of the type (which includes the namespace). Managed memory cannot be explicitly freed; instead, it is automatically garbage collected. Garbage collection addresses the problem of memory leaks by freeing the programmer of responsibility for releasing memory that is no longer needed in most cases. Code that retains references to objects longer than is required can still experience higher memory usage than necessary, however once the final reference to an object is released the memory is available for garbage collection.
POSIX standard library
Unions in C are related to structures and are defined as objects that may hold (at different times) objects of different types and sizes. Unlike structures, the components of a union all refer to the same location in memory. In this way, a union can be used at various times to hold different types of objects, without the need to create a separate object for each new type.
(Although char can represent any of C's "basic" characters, a wider type may be required for international character sets.) Most integer types have both signed and unsigned varieties, designated by the signed and unsigned keywords. Signed integer types may use a two's complement, ones' complement, or sign-and-magnitude representation. In many cases, there are multiple equivalent ways to designate the type; for example, signed short int and short are synonymous. The most common C library is the C standard library, which is specified by the ISO and ANSI C standards and comes with every C implementation (implementations which target limited environments such as embedded systems may provide only a subset of the standard library). This library supports stream input and output, memory allocation, mathematics, character strings, and time values. Several separate standard headers (for example, stdio.h) specify the interfaces for these and other standard library facilities.
It allows a programmer to specify characters that are otherwise difficult or impossible to specify in a literal. Variable pointers and references to a base class type in C++ can also refer to objects of any derived classes of that type. This allows arrays and other kinds of containers to hold pointers to objects of differing types (references cannot be directly held in containers). This enables dynamic (run-time) polymorphism, where the referred objects can behave differently, depending on their (actual, derived) types. C enables programmers to create efficient implementations of algorithms and data structures, because the layer of abstraction from hardware is thin, and its overhead is low, an important criterion for computationally intensive programs. For example, the GNU Multiple Precision Arithmetic Library, the GNU Scientific Library, Mathematica, and MATLAB are completely or partially written in C.
Edward C. Muehlfelt Jr. Obituaries channel3000.com - Channel3000.com - WISC-TV3
Edward C. Muehlfelt Jr. Obituaries channel3000.com.
Posted: Sun, 28 Apr 2024 18:56:00 GMT [source]
Nonetheless, a situation may arise where a copy of an object needs to be created when a pointer to a derived object is passed as a pointer to a base object. In such a case, a common solution is to create a clone() (or similar) virtual function that creates and returns a copy of the derived class when called. Structures aggregate the storage of multiple data items, of potentially differing data types, into one memory block referenced by a single variable.
The standard header file float.h defines the minimum and maximum values of the implementation's floating-point types float, double, and long double. It also defines other limits that are relevant to the processing of floating-point numbers. This declares the enum colors type; the int constants RED (whose value is 0), GREEN (whose value is one greater than RED, 1), BLUE (whose value is the given value, 5), and YELLOW (whose value is one greater than BLUE, 6); and the enum colors variable paint_color.
No comments:
Post a Comment